宣告式程式設計
以類似於無狀態小部件的方式編寫業務邏輯。
讓您的網路請求在必要時自動重新計算,並使您的邏輯易於重用、組合、維護。
輕鬆實現常見的 UI 模式
使用 Riverpod,只需幾行程式碼即可實現常見但複雜的 UI 模式,例如“下拉重新整理”、“即時搜尋”等。
工具準備就緒
Riverpod 透過將常見錯誤作為編譯錯誤來增強編譯器。 它還提供自定義 lint 規則和重構選項。 它甚至有一個用於生成文件的命令列。
特性
- ✅ Declarative programming
- ✅ Native network requests support
- ✅ Automatic loading/error handling
- ✅ Compile safety
- ✅ Type-safe query parameters
- ✅ Test ready
- ✅ Work in plain Dart (servers/CLI/...)
- ✅ Easily combinable states
- ✅ Built-in support for pull-to-refresh
- ✅ Custom lint rules
- ✅ Built-in refactorings
- ✅ Hot-reload support
- ✅ Logging
- ✅ Websocket support
- ✅ Documentation generator
隨處宣告共享狀態
無需在 main.dart
和 UI 檔案之間來回切換。
只要將共享狀態的程式碼放在它所屬位置上,無論是放在獨立的 package 中,還是需要它的 widget 旁,都不會丟失可測試性。
// A shared state that can be accessed by multiple widgets at the same time.
class Count extends _$Count {
int build() => 0;
void increment() => state++;
}
// Consumes the shared state and rebuild when it changes
class Title extends ConsumerWidget {
Widget build(BuildContext context, WidgetRef ref) {
final count = ref.watch(countProvider);
return Text('$count');
}
}
按需更新狀態和重繪 UI
不必在 build
方法中執行列表排序或過濾,也不必依賴高階的快取機制。
使用組合提供者 Provider
和 "families" 方法,在你真正需要的時候才去發起 HTTP 請求或者排序列表。
List<Todo> filteredTodos(Ref ref) {
// Providers can consume other providers using the "ref" object.
// With ref.watch, providers will automatically update if the watched values changes.
final List<Todo> todos = ref.watch(todosProvider);
final Filter filter = ref.watch(filterProvider);
switch (filter) {
case Filter.all:
return todos;
case Filter.completed:
return todos.where((todo) => todo.completed).toList();
case Filter.uncompleted:
return todos.where((todo) => !todo.completed).toList();
}
}
透過重構簡化日常工作
Riverpod 提供了多種重構提示, 例如 "Wrap widget in a Consumer" 等等。 請參閱 重構提示列表.
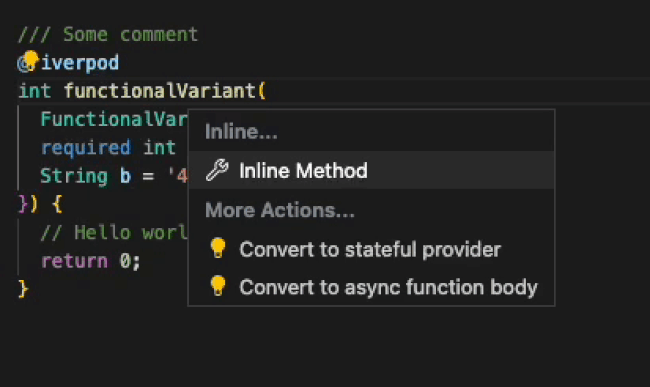
使用 lint 規則保持程式碼可維護
實現了 Riverpod 定製的 lint 新規則,並且還會不斷新增更多規則。這可確保您的程式碼保持最佳狀態。請參閱 lint 規則列表。
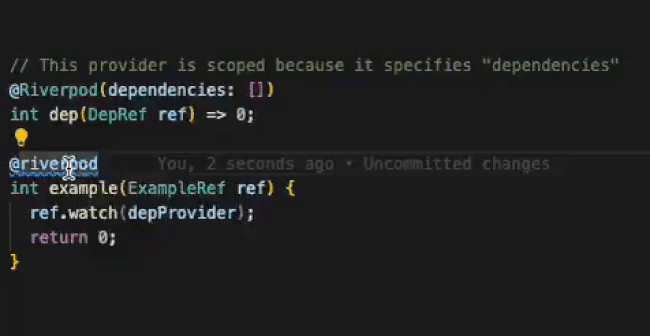
安全的讀取 providers
讀取 provider 永遠不會導致異常的狀態。如果你編寫了讀取一個 provider 所需的程式碼,你將得到一個符合預期的值。
這甚至適用於非同步載入的值。與 provider 相反,Riverpod 允許簡潔地處理 loading/error 狀態。
Future<Configuration> configurations(Ref ref) async {
final uri = Uri.parse('configs.json');
final rawJson = await File.fromUri(uri).readAsString();
return Configuration.fromJson(json.decode(rawJson));
}
class Example extends ConsumerWidget {
Widget build(BuildContext context, WidgetRef ref) {
final configs = ref.watch(configurationsProvider);
// Use pattern matching to safely handle loading/error states
return switch (configs) {
AsyncData(:final value) => Text('data: ${value.host}'),
AsyncError(:final error) => Text('error: $error'),
_ => const CircularProgressIndicator(),
};
}
}
在 DevTools 中檢查你的狀態
使用了 Riverpod,你的狀態將可以在 Flutter 自帶的 DevTools 中一目瞭然。
此外,一款全面的狀態檢查工具正在開發中……
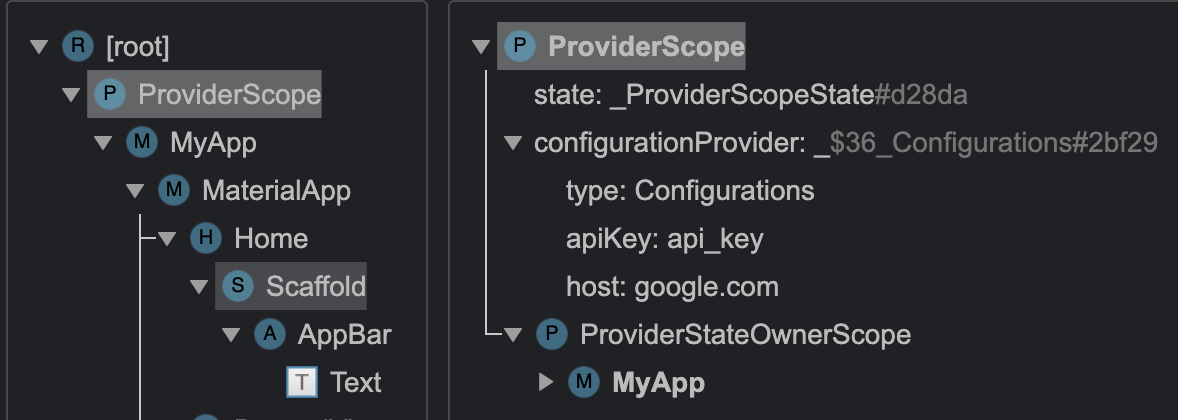